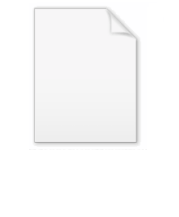
MuParser
Encyclopedia
muParser is an extensible high-performance math expression parser library written in C++
. It works by transforming a mathematical expression into bytecode
and precalculating constant parts of the expression.
It runs on both 32-bit
and 64-bit
architectures
and has been tested using Visual C++
and GCC
. The library is open source
and distributed under the MIT License
.
*The assignment operator is special since it changes one of its arguments and can only be applied to variables.
Example Code
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
. It works by transforming a mathematical expression into bytecode
Bytecode
Bytecode, also known as p-code , is a term which has been used to denote various forms of instruction sets designed for efficient execution by a software interpreter as well as being suitable for further compilation into machine code...
and precalculating constant parts of the expression.
It runs on both 32-bit
32-bit
The range of integer values that can be stored in 32 bits is 0 through 4,294,967,295. Hence, a processor with 32-bit memory addresses can directly access 4 GB of byte-addressable memory....
and 64-bit
64-bit
64-bit is a word size that defines certain classes of computer architecture, buses, memory and CPUs, and by extension the software that runs on them. 64-bit CPUs have existed in supercomputers since the 1970s and in RISC-based workstations and servers since the early 1990s...
architectures
Computer architecture
In computer science and engineering, computer architecture is the practical art of selecting and interconnecting hardware components to create computers that meet functional, performance and cost goals and the formal modelling of those systems....
and has been tested using Visual C++
Visual C++
Microsoft Visual C++ is a commercial , integrated development environment product from Microsoft for the C, C++, and C++/CLI programming languages...
and GCC
GNU Compiler Collection
The GNU Compiler Collection is a compiler system produced by the GNU Project supporting various programming languages. GCC is a key component of the GNU toolchain...
. The library is open source
Open source
The term open source describes practices in production and development that promote access to the end product's source materials. Some consider open source a philosophy, others consider it a pragmatic methodology...
and distributed under the MIT License
MIT License
The MIT License is a free software license originating at the Massachusetts Institute of Technology . It is a permissive license, meaning that it permits reuse within proprietary software provided all copies of the licensed software include a copy of the MIT License terms...
.
Overview
- Easy to use (only a few lines of code to evaluate an expression)
- Extremely fast
- User-defined operators (binary, postfix and infix)
- User-defined functions
- User-defined constants
- User-defined variables
- Custom value recognition callbacks
- Default implementation
- 26 predefined functions
- 15 predefined operators
- Supports numerical differentiation with respect to a given variable
- Assignment operator is supported
- Portability
- Project / makefiles for MSVC, mingw, autoconf, bcc
- ISO 14882 compliant code
- DLL version usable from every language able to use function exported in C-style
- Unit support (postfix operators as unit multipliers)
- Localization (of argument separator, decimal separator, thousands separator)
Built-in Functions
Name | Argument Count | Explanation |
sin |
1 | sine function |
cos |
1 | cosine function |
tan |
1 | tangens function |
asin |
1 | arcus sine function |
acos |
1 | arcus cosine function |
atan |
1 | arcus tangens function |
sinh |
1 | hyperbolic sine function |
cosh |
1 | hyperbolic cosine |
tanh |
1 | hyperbolic tangens function |
asinh |
1 | hyperbolic arcus sine function |
acosh |
1 | hyperbolic arcus tangens function |
atanh |
1 | hyperbolic arcur tangens function |
log2 |
1 | logarithm to the base 2 |
log10 |
1 | logarithm to the base 10 |
log |
1 | logarithm to the base 10 |
ln |
1 | logarithm to base e (2.71828...) |
exp |
1 | e raised to the power of x |
sqrt |
1 | square root of a value |
sign |
1 | sign function -1 if x<0; 1 if x>0 |
rint |
1 | round to nearest integer |
abs |
1 | absolute value |
if |
3 | if ... then ... else ... |
min |
var. | min of all arguments |
max |
var. | max of all arguments |
sum |
var. | sum of all arguments |
avg |
var. | mean value of all arguments |
Built-in operators
Operator | Meaning | Priority |
= |
assignment* | |
and |
logical and | 1 |
or |
logical or | 1 |
xor |
logical xor | 1 |
<= |
less or equal | 2 |
>= |
greater or equal | 2 |
!= |
not equal | 2 |
|
equal | 2 |
> |
greater than | 2 |
< |
less than | 2 |
+ |
addition | 3 |
- |
subtraction | 3 |
* |
multiplication | 4 |
/ |
division | 4 |
^ |
raise x to the power of y | 5 |
*The assignment operator is special since it changes one of its arguments and can only be applied to variables.
Example Code
#include
- include "muParser.h"
// Function callback
double MyFunction(double a_fVal)
{
return a_fVal*a_fVal;
}
// main program
int main(int argc, char* argv[])
{
using namespace mu;
try
{
double fVal = 1;
Parser p;
p.DefineVar("a", &fVal);
p.DefineFun("MyFunc", MyFunction);
p.SetExpr("MyFunc(a)*_pi+min(10,a)");
std::cout << p.Eval << std::endl;
}
catch (Parser::exception_type &e)
{
std::cout << e.GetMsg << std::endl;
}
return 0;
}