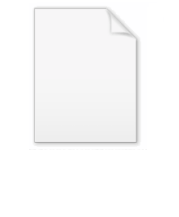
Comparison of programming languages (basic instructions)
Encyclopedia
Comparison of programming languages is a common topic of discussion among software engineer
s. Basic instructions of several programming language
s are compared here.
(« … ») are optional. indicates a necessary indent.
Integer
The standard constants int shorts and int lengths can be used to determine how many shorts and longs can be usefully prefixed to short int and long int. The actually size of the short int, int and long int is available as constants short max int, max int and long max int etc.
Commonly used for characters.
The ALGOL 68, C and C++ languages do not specify the exact width of the integer types "short", "int", "long", and (C99, C++11) "long long", so they are implementation-dependent. In C and C++ "short", "long", and "long long" types are required to be at least 16, 32, and 64 bits wide, respectively, but can be more. The "int" type is required to be at least as wide as "short" and at most as wide as "long", and is typically the width of the word size on the processor of the machine (i.e. on a 32-bit machine it is often 32 bits wide; on 64-bit machines it is often 64 bits wide). C99 and C++11 also defines the "[u]intN_t" exact-width types in the stdint.h header. See C syntax#Integral types for more information.
Perl 5 does not have distinct types. Integers, floating point numbers, strings, etc. are all considered "scalars".
PHP has two arbitrary-precision libraries. The BCMath library just uses strings as datatype. The GMP library uses an internal "resource" type.
The value of "n" is provided by the SELECTED_INT_KIND intrinsic function.
ALGOL 68G
's run time option --precision "number" can set precision for long long int's to the required "number" significant digits. The standard constants long long int width and long long max int can be used to determine actual precision.
Floating point
The standard constants real shorts and real lengths can be used to determine how many shorts and longs can be usefully prefixed to short real and long real. The actually size of the short real, real and long real is available as constants short max real, max real and long max real etc. With the constants short small real, small real and long small real available for each type's machine epsilon
.
declarations of single precision often are not honored
The value of "n" is provided by the SELECTED_REAL_KIND intrinsic function.
ALGOL 68G
's run time option --precision "number" can set precision for long long reals to the required "number" significant digits. The standard constants long long real width and long long max real can be used to determine actual precision.
Complex number
The value of "n" is provided by the SELECTED_REAL_KIND intrinsic function.
specifically, strings of arbitrary length and automatically managed.
This language represents a boolean as an integer where false is represented as a value of zero and true by a non-zero value.
All values evaluate to either true or false. Everything in TrueClass evaluates to true and everything in FalseClass evaluates to false.
This language does not have a separate character type. Characters are represented as strings of length 1.
Enumerations in this language are algebraic types with only nullary constructors
The value of "n" is provided by the SELECTED_INT_KIND intrinsic function.
Array
In most expressions (except the sizeof and & operators), values of array types in C are automatically converted to a pointer of its first argument. Also C's arrays can not be described in this format. See C syntax#Arrays.
The C-like "type x[]" works in Java, however "type[] x" is the preferred form of array declaration.
Subranges are used to define the bounds of the array.
JavaScript's array are a special kind of object.
Only classes are supported.
pair only
Although Perl doesn't have records, because Perl's type system allows different data types to be in an array, "hashes" (associative arrays) that don't have a variable index would effectively be the same as records.
Enumerations in this language are algebraic types with only nullary constructors
Pascal has declaration blocks. See Comparison of programming languages (basic instructions)#Functions.
Types are just regular objects, so you can just assign them.
In Perl, the "my" keyword scopes the variable into the block.
Technically, this does not declare name to be a mutable variable—in ML, all names can only be bound once; rather, it declares name to point to a "reference" data structure, which is a simple mutable cell. The data structure can then be read and written to using the ! and := operators, respectively.
A single instruction can be written on the same line following the colon. Multiple instructions are grouped together in a block which starts on a newline (The indentation in required). The conditional expression syntax does not follow this rule.
This is pattern matching
and is similar to select case but not the same. It is usually used to deconstruct algebraic data type
s.
In languages of the Pascal family, the semicolon is not part of the statement. It is a separator between statements, not a terminator.
"step n" is used to change the loop interval. If "step" is omitted, then the loop interval is 1.
Exceptions
Common Lisp allows
Pascal has declaration blocks. See Comparison of programming languages (basic instructions)#Functions.
label must be a number between 1 and 99999.
Functions
See reflection
for calling and declaring functions by strings.
Pascal requires "forward;" for forward declarations.
Eiffel allows the specification of an application's root class and feature.
In Fortran, function/subroutine parameters are called arguments (since PARAMETER is a language keyword); the CALL keyword is required for subroutines.
Type conversion
Where string is a signed decimal number:
JavaScript only uses floating point numbers so there are some technicalities.
Perl doesn't have separate types. Strings and numbers are interchangeable.
Standard Stream I/O
Algol 68 additionally as the "unformatted" transput routines: read, write, get and put.
gets(x) and fgets(x, length, stdin) read unformatted text from stdin. Use of gets is not recommended.
puts(x) and fputs(x, stdout) write unformatted text to stdout.
fputs(x, stderr) writes unformatted text to stderr
INPUT_UNIT, OUTPUT_UNIT, ERROR_UNIT are defined in the ISO_FORTRAN_ENV module.
The command-line arguments in Visual Basic are not separated. A split function Split(string) is required for separating them.
Compiler-dependent extension.
Software engineer
A software engineer is an engineer who applies the principles of software engineering to the design, development, testing, and evaluation of the software and systems that make computers or anything containing software, such as computer chips, work.- Overview :...
s. Basic instructions of several programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s are compared here.
Conventions of this article
The bold is the literal code. The non-bold is interpreted by the reader. Statements in guillemetsGuillemets
Guillemets , also called angle quotes, are line segments, pointed as if arrows , sometimes forming a complementary set of punctuation marks used as a form of quotation mark....
(« … ») are optional. indicates a necessary indent.
IntegerInteger (computer science)In computer science, an integer is a datum of integral data type, a data type which represents some finite subset of the mathematical integers. Integral data types may be of different sizes and may or may not be allowed to contain negative values....
s
8 bit (byte Byte The byte is a unit of digital information in computing and telecommunications that most commonly consists of eight bits. Historically, a byte was the number of bits used to encode a single character of text in a computer and for this reason it is the basic addressable element in many computer... ) |
16 bit (short integer) | 32 bit | 64 bit (long integer) | Word size | Arbitrarily precise (bignum Arbitrary-precision arithmetic In computer science, arbitrary-precision arithmetic indicates that calculations are performed on numbers whose digits of precision are limited only by the available memory of the host system. This contrasts with the faster fixed-precision arithmetic found in most ALU hardware, which typically... ) |
||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Signed | Unsigned | Signed | Unsigned | Signed | Unsigned | Signed | Unsigned | Signed | Unsigned | ||
ALGOL 68 ALGOL 68 ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a... (variable-width) |
short short int | rowspan=2 | short int | rowspan=2 | int | rowspan=2 | long int | rowspan=2 | int | long long int | |
bytes & bits | |||||||||||
C C (programming language) C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system.... (C99 C99 C99 is a modern dialect of the C programming language. It extends the previous version with new linguistic and library features, and helps implementations make better use of available computer hardware and compiler technology.-History:... fixed-width) |
int8_t | uint8_t | int16_t | uint16_t | int32_t | uint32_t | int64_t | uint64_t | int | unsigned int | rowspan=5 |
C++ C++ C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell... (C++11 fixed-width) |
|||||||||||
C C (programming language) C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system.... (C99 C99 C99 is a modern dialect of the C programming language. It extends the previous version with new linguistic and library features, and helps implementations make better use of available computer hardware and compiler technology.-History:... variable-width) |
signed char | unsigned char | short | unsigned short | long | unsigned long | long long | unsigned long long | |||
C++ C++ C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell... (C++11 variable-width) |
|||||||||||
Objective-C Objective-C Objective-C is a reflective, object-oriented programming language that adds Smalltalk-style messaging to the C programming language.Today, it is used primarily on Apple's Mac OS X and iOS: two environments derived from the OpenStep standard, though not compliant with it... |
signed char | unsigned char | short | unsigned short | long | unsigned long | long long | unsigned long long | int or NSInteger | unsigned int or NSUInteger | |
C# | sbyte | byte | short | ushort | int | uint | long | ulong | rowspan=2 colspan=2 | System.Numerics.BigInteger (.NET 4.0) | |
Java Java (programming language) Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities... |
byte | char | java.math.BigInteger | ||||||||
Go Go (programming language) Go is a compiled, garbage-collected, concurrent programming language developed by Google Inc.The initial design of Go was started in September 2007 by Robert Griesemer, Rob Pike, and Ken Thompson. Go was officially announced in November 2009. In May 2010, Rob Pike publicly stated that Go was being... |
int8 | uint8 or byte | int16 | uint16 | int32 | uint32 | int64 | uint64 | int | uint | big.Int |
D D (programming language) The D programming language is an object-oriented, imperative, multi-paradigm, system programming language created by Walter Bright of Digital Mars. It originated as a re-engineering of C++, but even though it is mainly influenced by that language, it is not a variant of C++... |
byte | ubyte | short | ushort | int | uint | long | ulong | BigInt | ||
Common Lisp Common Lisp Common Lisp, commonly abbreviated CL, is a dialect of the Lisp programming language, published in ANSI standard document ANSI INCITS 226-1994 , . From the ANSI Common Lisp standard the Common Lisp HyperSpec has been derived for use with web browsers... |
bignum | ||||||||||
Scheme | |||||||||||
Pascal Pascal (programming language) Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal... (FPC Free Pascal Free Pascal Compiler is a free Pascal and Object Pascal compiler.In addition to its own Object Pascal dialect, Free Pascal supports, to varying degrees, the dialects of several other compilers, including those of Turbo Pascal, Delphi, and some historical Macintosh compilers... ) |
shortint | byte | smallint | word | longint | longword | int64 | qword | integer | cardinal | |
Visual Basic Visual Basic Visual Basic is the third-generation event-driven programming language and integrated development environment from Microsoft for its COM programming model... |
Byte | Integer | Long | colspan=2 | rowspan=2 colspan=2 | rowspan=1 | |||||
Visual Basic .NET Visual Basic .NET Visual Basic .NET , is an object-oriented computer programming language that can be viewed as an evolution of the classic Visual Basic , which is implemented on the .NET Framework... |
SByte | Short | UShort | Integer | UInteger | Long | ULong | System.Numerics.BigInteger (.NET 4.0) | |||
Python Python (programming language) Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive... 2.x |
colspan=2 | colspan=2 | colspan=2 | colspan=2 | int | long | |||||
Python 3.x | colspan=2 | colspan=2 | colspan=2 | colspan=2 | colspan=2 | int | |||||
S-Lang | colspan=2 | colspan=2 | colspan=2 | colspan=2 | colspan=2 | ||||||
Fortran Fortran Fortran is a general-purpose, procedural, imperative programming language that is especially suited to numeric computation and scientific computing... |
INTEGER(KIND = n) | INTEGER(KIND = n) | INTEGER(KIND = n) | INTEGER(KIND = n) | |||||||
PHP PHP PHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document... |
colspan=2 | colspan=2 | int | colspan=2 | colspan=2 | ||||||
Perl Perl Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular... 5 |
colspan=2 | colspan=2 | colspan=2 | colspan=2 | colspan=2 | Math::BigInt | |||||
Perl 6 Perl 6 Perl 6 is a major revision to the Perl programming language. It is still in development, as a specification from which several interpreter and compiler implementations are being written. It is introducing elements of many modern and historical languages. Perl 6 is intended to have many... |
int8 | uint8 | int16 | uint16 | int32 | uint32 | int64 | uint64 | Int | colspan=2 | |
Ruby Ruby (programming language) Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto... |
colspan=2 | colspan=2 | colspan=2 | colspan=2 | Fixnum | Bignum | |||||
Windows PowerShell Windows PowerShell Windows PowerShell is Microsoft's task automation framework, consisting of a command-line shell and associated scripting language built on top of, and integrated with the .NET Framework... |
colspan=2 | colspan=2 | colspan=2 | colspan=2 | colspan=2 | ||||||
OCaml | colspan=2 | colspan=2 | int32 | int64 | int or nativeint |
open Big_int;; big_int |
|||||
F# | sbyte | byte | int16 | uint16 | int32 or int | uint32 | uint64 | nativeint | unativeint | bigint | |
Standard ML Standard ML Standard ML is a general-purpose, modular, functional programming language with compile-time type checking and type inference. It is popular among compiler writers and programming language researchers, as well as in the development of theorem provers.SML is a modern descendant of the ML... |
Word8.word | colspan=2 | Int32.int | Word32.word | Int64.int | Word64.word | int | word | LargeInt.int or IntInf.int |
||
Haskell Haskell (programming language) Haskell is a standardized, general-purpose purely functional programming language, with non-strict semantics and strong static typing. It is named after logician Haskell Curry. In Haskell, "a function is a first-class citizen" of the programming language. As a functional programming language, the... (GHC Glasgow Haskell Compiler The Glorious Glasgow Haskell Compilation System, more commonly known as the Glasgow Haskell Compiler or GHC, is an open source native code compiler for the functional programming language Haskell. The lead developers are Simon Peyton Jones and Simon Marlow... ) |
«import Int» Int8 |
«import Word» Word8 |
«import Int» Int16 |
«import Word» Word16 |
«import Int» Int32 |
«import Word» Word32 |
«import Int» Int64 |
«import Word» Word64 |
Int | «import Word» Word |
Integer |
Eiffel Eiffel (programming language) Eiffel is an ISO-standardized, object-oriented programming language designed by Bertrand Meyer and Eiffel Software. The design of the language is closely connected with the Eiffel programming method... |
INTEGER_8 | NATURAL_8 | INTEGER_16 | NATURAL_16 | INTEGER_32 | NATURAL_32 | INTEGER_64 | NATURAL_64 | INTEGER | NATURAL |
The standard constants int shorts and int lengths can be used to determine how many shorts and longs can be usefully prefixed to short int and long int. The actually size of the short int, int and long int is available as constants short max int, max int and long max int etc.
Commonly used for characters.
The ALGOL 68, C and C++ languages do not specify the exact width of the integer types "short", "int", "long", and (C99, C++11) "long long", so they are implementation-dependent. In C and C++ "short", "long", and "long long" types are required to be at least 16, 32, and 64 bits wide, respectively, but can be more. The "int" type is required to be at least as wide as "short" and at most as wide as "long", and is typically the width of the word size on the processor of the machine (i.e. on a 32-bit machine it is often 32 bits wide; on 64-bit machines it is often 64 bits wide). C99 and C++11 also defines the "[u]intN_t" exact-width types in the stdint.h header. See C syntax#Integral types for more information.
Perl 5 does not have distinct types. Integers, floating point numbers, strings, etc. are all considered "scalars".
PHP has two arbitrary-precision libraries. The BCMath library just uses strings as datatype. The GMP library uses an internal "resource" type.
The value of "n" is provided by the SELECTED_INT_KIND intrinsic function.
ALGOL 68G
ALGOL 68G
ALGOL68G or Algol 68 Genie is a recent ALGOL 68 compiler-interpreter. ALGOL68G is a nearly full implementation of ALGOL 68 as defined by the Revised Report and also implements partial parametrisation, which is an extension of ALGOL 68. After successful parsing of an entire source program, the...
's run time option --precision "number" can set precision for long long int's to the required "number" significant digits. The standard constants long long int width and long long max int can be used to determine actual precision.
Floating pointFloating pointIn computing, floating point describes a method of representing real numbers in a way that can support a wide range of values. Numbers are, in general, represented approximately to a fixed number of significant digits and scaled using an exponent. The base for the scaling is normally 2, 10 or 16...
Single precision | Double precision Double precision In computing, double precision is a computer number format that occupies two adjacent storage locations in computer memory. A double-precision number, sometimes simply called a double, may be defined to be an integer, fixed point, or floating point .Modern computers with 32-bit storage locations... |
Processor dependent | |
---|---|---|---|
ALGOL 68 | real | long real | short real etc. & long long real etc. |
C | float | double | rowspan=3 |
Objective-C | |||
C++ (STL) | |||
C# | float | rowspan=3 | |
Java | |||
Go | float32 | float64 | |
D | float | double | real |
Common Lisp | |||
Scheme | |||
Pascal (Free Pascal) | single | double | real |
Visual Basic | Single | Double | rowspan=2 |
Visual Basic .NET | |||
Python | rowspan=2 | float | |
JavaScript | Number | ||
S-Lang | |||
Fortran | REAL(KIND = n) | ||
PHP | float | ||
Perl | |||
Perl 6 | num32 | num64 | Num |
Ruby | Float | rowspan=3 | |
Windows PowerShell | |||
OCaml | float | ||
F# | float32 | ||
Standard ML | real | ||
Haskell (GHC) | Float | Double | |
Eiffel | REAL_32 | REAL_64 |
The standard constants real shorts and real lengths can be used to determine how many shorts and longs can be usefully prefixed to short real and long real. The actually size of the short real, real and long real is available as constants short max real, max real and long max real etc. With the constants short small real, small real and long small real available for each type's machine epsilon
Machine epsilon
Machine epsilon gives an upper bound on the relative error due to rounding in floating point arithmetic. This value characterizes computer arithmetic in the field of numerical analysis, and by extension in the subject of computational science...
.
declarations of single precision often are not honored
The value of "n" is provided by the SELECTED_REAL_KIND intrinsic function.
ALGOL 68G
ALGOL 68G
ALGOL68G or Algol 68 Genie is a recent ALGOL 68 compiler-interpreter. ALGOL68G is a nearly full implementation of ALGOL 68 as defined by the Revised Report and also implements partial parametrisation, which is an extension of ALGOL 68. After successful parsing of an entire source program, the...
's run time option --precision "number" can set precision for long long reals to the required "number" significant digits. The standard constants long long real width and long long max real can be used to determine actual precision.
Complex numberComplex numberA complex number is a number consisting of a real part and an imaginary part. Complex numbers extend the idea of the one-dimensional number line to the two-dimensional complex plane by using the number line for the real part and adding a vertical axis to plot the imaginary part...
s
Integer | Single precision | Double precision | Half and Quadruple precision etc. | |
---|---|---|---|---|
ALGOL 68 | compl | long compl etc. | short compl etc. & long long compl etc. | |
C (C99) | float complex | double complex | rowspan=12 | |
C++ (STL) | «std::»complex |
«std::»complex |
||
C# | System.Numerics.Complex (.Net 4.0) |
|||
Java | ||||
Go | complex64 | complex128 | ||
D | cfloat | cdouble | ||
Objective-C | ||||
Common Lisp | ||||
Scheme | ||||
Pascal | ||||
Visual Basic | ||||
Visual Basic .NET | System.Numerics.Complex (.Net 4.0) |
|||
Perl | Math::Complex | |||
Perl 6 | complex64 | complex128 | Complex | |
Python | complex | rowspan=12 | ||
JavaScript | ||||
S-Lang | ||||
Fortran | COMPLEX(KIND = n) | |||
Ruby | Complex | Complex | ||
Windows PowerShell | ||||
OCaml | Complex.t | |||
F# | System.Numerics.Complex (.Net 4.0) |
|||
Standard ML | ||||
Haskell (GHC) | Complex.Complex Float | Complex.Complex Double | ||
Eiffel |
The value of "n" is provided by the SELECTED_REAL_KIND intrinsic function.
Other variable types
Text | Boolean Boolean datatype In computer science, the Boolean or logical data type is a data type, having two values , intended to represent the truth values of logic and Boolean algebra... |
Enumeration Enumerated type In computer programming, an enumerated type is a data type consisting of a set of named values called elements, members or enumerators of the type. The enumerator names are usually identifiers that behave as constants in the language... |
Object Object (computer science) In computer science, an object is any entity that can be manipulated by the commands of a programming language, such as a value, variable, function, or data structure... /Universal Top type The top type in type theory, commonly abbreviated as top or by the down tack symbol , is the universal type—that type which contains every possible object in the type system of interest. The top type is sometimes called the universal supertype as all other types in any given type system are... |
||
---|---|---|---|---|---|
Character Character (computing) In computer and machine-based telecommunications terminology, a character is a unit of information that roughly corresponds to a grapheme, grapheme-like unit, or symbol, such as in an alphabet or syllabary in the written form of a natural language.... |
String String (computer science) In formal languages, which are used in mathematical logic and theoretical computer science, a string is a finite sequence of symbols that are chosen from a set or alphabet.... |
||||
ALGOL 68 | char | string & bytes | bool & bits | - User defined | |
C (C99) | char wchar_t |
bool | enum «name» {item1, item2, ... }; | void Void type The void type, in several programming languages derived from C and Algol68, is the type for the result of a function that returns normally, but does not provide a result value to its caller. Usually such functions are called for their side effects, such as performing some task or writing to their... * |
|
C++ (STL) | «std::»string | ||||
Objective-C | unichar | NSString * | BOOL | id | |
C# | char | string | bool | enum name {item1, item2, ... } | object |
Java | String | boolean | Object | ||
Go | byte or int | string | bool | const (
item2 ... |
interface{} |
D | char | string | bool | enum name {item1, item2, ... } | std.variant.Variant |
Common Lisp | |||||
Scheme | |||||
Pascal (ISO) | char | boolean | (item1, item2, ...) | ||
Object Pascal (Delphi) | string | variant | |||
Visual Basic | String | Boolean | Enum name
item2 ... |
Variant Variant type Variant is a data type in certain programming languages, particularly Visual Basic and C++ when using the Component Object Model.In Visual Basic the Variant data type is a tagged union that can be used to represent any other data type except fixed-length string type and... |
|
Visual Basic .NET | Char | Object | |||
Python | str | bool | object | ||
JavaScript | String | Boolean | Object | ||
S-Lang | |||||
Fortran | CHARACTER(LEN = *) | CHARACTER(LEN = :), allocatable | LOGICAL(KIND = n) | CLASS(*) | |
PHP | string | bool | object | ||
Perl | |||||
Perl 6 | Char | Str | Bool | enum name <item1 item2 ...> or enum name <<:item1(value) :item2(value) ...>> |
Mu |
Ruby | String | Object | Object | ||
Windows PowerShell | |||||
OCaml | char | string | bool | ||
F# | type name = item1 = value |
obj | |||
Standard ML | |||||
Haskell (GHC) | Char | String | Bool | ||
Eiffel | CHARACTER | STRING | BOOLEAN | ANY |
specifically, strings of arbitrary length and automatically managed.
This language represents a boolean as an integer where false is represented as a value of zero and true by a non-zero value.
All values evaluate to either true or false. Everything in TrueClass evaluates to true and everything in FalseClass evaluates to false.
This language does not have a separate character type. Characters are represented as strings of length 1.
Enumerations in this language are algebraic types with only nullary constructors
The value of "n" is provided by the SELECTED_INT_KIND intrinsic function.
ArrayArray data typeIn computer science, an array type is a data type that is meant to describe a collection of elements , each selected by one or more indices that can be computed at run time by the program. Such a collection is usually called an array variable, array value, or simply array...
fixed size array | dynamic size array | |||
---|---|---|---|---|
one-dimensional array | multi-dimensional array | one-dimensional array | multi-dimensional array | |
ALGOL 68 | [first:last]«modename» or simply: [size]«modename» | [first1:last1,first2:last2]«modename» or [first1:last1][first2:last2]«modename» etc. | flex[first:last]«modename» or simply: flex[size]«modename» | flex[first1:last1,first2:last2]«modename» or flex[first1:last1]flex[first2:last2]«modename» etc. |
C (C99) | ||||
C++ (STL) | «std::»array<type, size>(C++11) | «std::»vector<type> | ||
C# | type[] | type[,,...] | System.Collections.ArrayList or System.Collections.Generic.List<type> |
|
Java | type[] | type[][]... | ArrayList or ArrayList<type> | |
D | type[size] | type[size1][size2] | type[] | |
Go | [size]type | [size1][size2]...type | vector.Vector | |
Objective-C | NSArray | NSMutableArray | ||
JavaScript | Array | |||
Common Lisp | ||||
Scheme | ||||
Pascal | array[first..last] of type | array[first1..last1] of array[first2..last2] ... of type or array[first1..last1, first2..last2, ...] of type |
||
Object Pascal (Delphi) | array of type | array of array ... of type | ||
Visual Basic | ||||
Visual Basic .NET | System.Collections.ArrayList or System.Collections.Generic.List(Of type) |
|||
Python | list | |||
S-Lang | ||||
Fortran | type :: name(size) | type :: name(size1, size2,...) | type, ALLOCATABLE :: name(:) | type, ALLOCATABLE :: name(:,:,...) |
PHP | array | |||
Perl | ||||
Perl 6 | Array[type] or Array of type | |||
Ruby | Array | |||
Windows PowerShell | type[] | type[,,...] | ||
OCaml | type array | type array ... array | ||
F# | type [] or type array | type [,,...] | System.Collections.ArrayList or System.Collections.Generic.List<type> |
|
Standard ML | type vector or type array | |||
Haskell (GHC) |
In most expressions (except the sizeof and & operators), values of array types in C are automatically converted to a pointer of its first argument. Also C's arrays can not be described in this format. See C syntax#Arrays.
The C-like "type x[]" works in Java, however "type[] x" is the preferred form of array declaration.
Subranges are used to define the bounds of the array.
JavaScript's array are a special kind of object.
Other types
Simple composite types | Algebraic data type Algebraic data type In computer programming, particularly functional programming and type theory, an algebraic data type is a datatype each of whose values is data from other datatypes wrapped in one of the constructors of the datatype. Any wrapped datum is an argument to the constructor... s |
Unions Union (computer science) In computer science, a union is a value that may have any of several representations or formats; or a data structure that consists of a variable which may hold such a value. Some programming languages support special data types, called union types, to describe such values and variables... |
||
---|---|---|---|---|
Records Record (computer science) In computer science, a record is an instance of a product of primitive data types called a tuple. In C it is the compound data in a struct. Records are among the simplest data structures. A record is a value that contains other values, typically in fixed number and sequence and typically indexed... |
Tuple Tuple In mathematics and computer science, a tuple is an ordered list of elements. In set theory, an n-tuple is a sequence of n elements, where n is a positive integer. There is also one 0-tuple, an empty sequence. An n-tuple is defined inductively using the construction of an ordered pair... expression |
|||
ALGOL 68 | struct (modename «fieldname», ...); | Required types and operators can be user defined | union (modename, ...); | |
C (C99) | struct «name» {type name;...}; | rowspan=2 | rowspan=3 | union {type name;...}; |
Objective-C | ||||
C++ | ||||
C# | struct name {type name;...} | rowspan=3 | ||
Java | ||||
JavaScript | ||||
D | struct name {type name;...} | std.variant.Algebraic!(type,...) | union {type name;...} | |
Go | struct {
... |
|||
Common Lisp | (cons val1 val2) | |||
Scheme | ||||
Pascal | record
...end |
record value: (types); ...end |
||
Visual Basic | ||||
Visual Basic .NET | Structure name ... End Structure |
|||
Python | «(»val1, val2, val3, ... «)» | |||
S-Lang | struct {name [=value], ...} | |||
Fortran | TYPE name ... END TYPE |
|||
PHP | ||||
Perl | rowspan=3 | |||
Perl 6 | ||||
Ruby | OpenStruct.new({:name => value}) | |||
Windows PowerShell | ||||
OCaml | type name = {«mutable» name : type;...} | «(»val1, val2, val3, ... «)» | type name = Foo «of type» |
rowspan=4 |
F# | ||||
Standard ML | type name = {name : type,...} | (val1, val2, val3, ... ) | datatype name = Foo «of type» |
|
Haskell | data Name = Constr {name :: type,...} | data Name = Foo «types» |
Only classes are supported.
struct
s in C++ are actually classes, but POD objects are in fact records.pair only
Although Perl doesn't have records, because Perl's type system allows different data types to be in an array, "hashes" (associative arrays) that don't have a variable index would effectively be the same as records.
Enumerations in this language are algebraic types with only nullary constructors
Variable and Constant Declarations
variable | constant | type synonym | |
---|---|---|---|
ALGOL 68 ALGOL 68 ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a... |
modename name «:= initial_value»; | modename name = value; | mode Typedef typedef is a keyword in the C and C++ programming languages. The purpose of typedef is to assign alternative names to existing types, most often those whose standard declaration is cumbersome, potentially confusing, or likely to vary from one implementation to another.Under C convention , types... synonym = modename; |
C (C99) | type name «= initial_value»; | enum{ name = value }; | typedef Typedef typedef is a keyword in the C and C++ programming languages. The purpose of typedef is to assign alternative names to existing types, most often those whose standard declaration is cumbersome, potentially confusing, or likely to vary from one implementation to another.Under C convention , types... type synonym; |
Objective-C | |||
C++ | const type name = value; | ||
C# | type name «= initial_value»; or var name = value; |
const type name = value; or readonly type name = value; |
using synonym = type; |
D | type name «= initial_value»; or auto name = value; |
const type name = value; or immutable type name = value; |
alias type synonym; |
Java | type name «= initial_value»; | final type name = value; | rowspan=2 |
JavaScript | var name «= initial_value»; | const name = value; | |
Go | var name type «= initial_value» or name := initial_value |
const name «type» = initial_value | type synonym type |
Common Lisp | (defparameter name initial_value) or (defvar name initial_value) or (setf (symbol-value |
(defconstant name value) | (deftype synonym |
Scheme | (define name initial_value) | ||
Pascal | name: type «= initial_value» | name = value | synonym = type |
Visual Basic | Dim name As type | Const name As type = value | |
Visual Basic .NET | Dim name As type«= initial_value» | Imports synonym = type | |
Python | name = initial_value | synonym = type | |
S-Lang | name = initial_value; | typedef struct {...} typename | |
Fortran | type name | type, PARAMETER :: name = value | |
PHP | $name = initial_value; | define("name", value); const name = value (5.3+) |
rowspan=2 |
Perl | «my» $name «= initial_value»; | use constant name => value; | |
Perl 6 | «my «type»» $name «= initial_value»; | «my «type»» constant name = value; | ::synonym ::= type |
Ruby | name = initial_value | Name = value | synonym = type |
Windows PowerShell | «[type]» $name = initial_value | ||
OCaml | let name «: type ref» = ref value | let name «: type» = value | type synonym = type |
F# | let mutable name «: type» = value | ||
Standard ML | val name «: type ref» = ref value | val name «: type» = value | |
Haskell | «name::type;» name = value | type Synonym = type | |
Forth | VARIABLE name (in some systems use value VARIABLE name instead) | value CONSTANT name |
Pascal has declaration blocks. See Comparison of programming languages (basic instructions)#Functions.
Types are just regular objects, so you can just assign them.
In Perl, the "my" keyword scopes the variable into the block.
Technically, this does not declare name to be a mutable variable—in ML, all names can only be bound once; rather, it declares name to point to a "reference" data structure, which is a simple mutable cell. The data structure can then be read and written to using the ! and := operators, respectively.
Conditional statements
if | else if | select case Switch statement In computer programming, a switch, case, select or inspect statement is a type of selection control mechanism that exists in most imperative programming languages such as Pascal, Ada, C/C++, C#, Java, and so on. It is also included in several other types of languages... |
conditional expression | |
---|---|---|---|---|
ALGOL 68 ALGOL 68 ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a... & "brief form" |
if condition then statements «else statements» fi | if condition then statements elif condition then statements fi | case switch in statements, statements«,... out statements» esac | ( condition | valueIfTrue | valueIfFalse ) |
statements «| statements» ) | statements |: condition | statements ) | statements,... «| statements» ) | ||
C (C99) | if (condition) {instructions} «else {instructions}» |
if (condition) {instructions} else if (condition) {instructions} ... «else {instructions}» |
switch (variable) {
... «default: instructions»} |
condition ? ?: In computer programming, ?: is a ternary operator that is part of the syntax for a basic conditional expression in several programming languages... valueIfTrue : valueIfFalse |
Objective-C | ||||
C++ (STL) | ||||
D | ||||
Java | ||||
JavaScript | ||||
PHP | ||||
C# | switch (variable) {
... «default: instructions; «jump statement;»» } |
|||
Windows PowerShell | if (condition) { instructions } elseif (condition) { instructions } ... «else { instructions }» |
switch (variable) { case1 { instructions «break;» } ... «default { instructions }»} | ||
Go | if condition {instructions} «else {instructions}» |
if condition {instructions} else if condition {instructions} ... «else {instructions}» or switch {
... «default: instructions»} |
switch variable {
... «default: instructions»} |
|
Perl | if (condition) {instructions} «else {instructions}» or unless (notcondition) {instructions} «else {instructions}» |
if (condition) {instructions} elsif (condition) {instructions} ... «else {instructions}» or unless (notcondition) {instructions} elsif (condition) {instructions} ... «else {instructions}» |
use feature "switch"; ... given (variable) {
... «default { instructions }»} |
condition ? valueIfTrue : valueIfFalse |
Perl 6 | if condition {instructions} «else {instructions}» or unless notcondition {instructions} |
if condition {instructions} elsif condition {instructions} ... «else {instructions} |
given variable {
... «default { instructions }»} |
condition ?? valueIfTrue !! valueIfFalse |
Ruby | if condition
|
if condition
«else
|
case variable when case1
«else
|
condition ? valueIfTrue : valueIfFalse |
Common Lisp | (when condition
(unless condition
(if condition
|
(cond (condition1 instructions)
|
(case expression
|
(if condition valueIfTrue valueIfFalse) |
Scheme | (when conditioninstructions) or (if condition (begin instructions) «(begin instructions)») |
(cond (condition1 instructions) (condition2 instructions) ... «(else instructions)») | (case (variable) ((case1) instructions) ((case2) instructions) ... «(else instructions)») | |
Pascal | if condition then begin
«else begin
|
if condition then begin
else if condition then begin
... «else begin
|
case variable of
... «else: instructions» |
|
Visual Basic | If condition Then
|
If condition Then
«Else
|
Select Case variable Case case1
«Case Else
|
IIf IIf In computing, IIf is a function in several editions of the Visual Basic programming language, related languages such as ColdFusion Markup Language , and on spreadsheets that returns one of its three parameters based on the evaluation of one of the other parameters... (condition, valueIfTrue, valueIfFalse) |
Visual Basic .NET | If(condition, valueIfTrue, valueIfFalse) | |||
Python | if condition : instructions «else: instructions» |
if condition : instructions elif condition : instructions ... «else: instructions» |
valueIfTrue if condition else valueIfFalse (Python 2.5+) |
|
S-Lang | if (condition) { instructions } «else { instructions }» | if (condition) { instructions } else if (condition) { instructions } ... «else { instructions }» | switch (variable) { case case1: instructions } { case case2: instructions } ... | |
Fortran | IF (condition) THEN |
IF (condition) THEN ELSE |
SELECT CASE(variable)
CASE DEFAULT |
|
Forth | condition IF instructions « ELSE instructions» THEN | condition IF instructions ELSE condition IF instructions THEN THEN | value CASE case OF instructions ENDOF case OF instructions ENDOF default instructions ENDCASE |
condition IF valueIfTrue ELSE valueIfFalse THEN |
OCaml | if condition then begin instructions end «else begin instructions end» | if condition then begin instructions end else if condition then begin instructions end ... «else begin instructions end» | match value with
... « |
if condition then valueIfTrue else valueIfFalse |
F# | if condition then instructions «else instructions» |
if condition then instructions elif condition then instructions ... «else instructions» |
||
Standard ML | if condition then «(»instructions «)» else «(» instructions «)» |
if condition then «(»instructions «)» else if condition then «(» instructions «)» ... else «(» instructions «)» |
case value of
... « |
|
Haskell (GHC) | if condition then expression else expression or when condition (do instructions) or unless notcondition (do instructions) |
case value of {
pattern2 -> expression; ... «_ -> expression» |
||
if | else if | select case Switch statement In computer programming, a switch, case, select or inspect statement is a type of selection control mechanism that exists in most imperative programming languages such as Pascal, Ada, C/C++, C#, Java, and so on. It is also included in several other types of languages... |
conditional expression |
A single instruction can be written on the same line following the colon. Multiple instructions are grouped together in a block which starts on a newline (The indentation in required). The conditional expression syntax does not follow this rule.
This is pattern matching
Pattern matching
In computer science, pattern matching is the act of checking some sequence of tokens for the presence of the constituents of some pattern. In contrast to pattern recognition, the match usually has to be exact. The patterns generally have the form of either sequences or tree structures...
and is similar to select case but not the same. It is usually used to deconstruct algebraic data type
Algebraic data type
In computer programming, particularly functional programming and type theory, an algebraic data type is a datatype each of whose values is data from other datatypes wrapped in one of the constructors of the datatype. Any wrapped datum is an argument to the constructor...
s.
In languages of the Pascal family, the semicolon is not part of the statement. It is a separator between statements, not a terminator.
Loop statements
while While loop In most computer programming languages, a while loop is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. The while loop can be thought of as a repeating if statement.... |
do while Do while loop In most computer programming languages, a do while loop, sometimes just called a do loop, is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. Note though that unlike most languages, Fortran's do loop is actually analogous to the for loop.The... |
for i = first to last For loop In computer science a for loop is a programming language statement which allows code to be repeatedly executed. A for loop is classified as an iteration statement.... |
foreach Foreach For each is a computer language idiom for traversing items in a collection. Foreach is usually used in place of a standard for statement. Unlike other for loop constructs, however, foreach loops usually maintain no explicit counter: they essentially say "do this to everything in this set",... |
|
---|---|---|---|---|
ALGOL 68 ALGOL 68 ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a... |
«for index» «from first» «by increment» «to last» «while condition» do statements od | for key «to upb list» do «typename val=list[key];» statements od | ||
«while condition» do statements od |
«while statements; condition» do statements od |
«for index» «from first» «by increment» «to last» do statements od | ||
C (C99) | while (condition) { instructions } | do { instructions } while (condition) | for («type» i = first; i <= last; ++i) { instructions } | |
Objective-C | for (type item in set) { instructions } | |||
C++ (STL) | «std::»for_each(start, end, function) (C++11) for (type item : set) { instructions } |
|||
C# | foreach (type item in set) { instructions } | |||
Java | for (type item : set) { instructions } | |||
JavaScript | for (var i = first; i <= last; i++) { instructions } | for (var index in set) { instructions } or for each (var item in set) { instructions } (JS 1.6+) |
||
PHP | foreach (range(first, last-1) as $i) { instructions } or for ($i = first; $i <= last; $i++) { instructions } |
foreach (set as item) { instructions } or foreach (set as key => item) { instructions } |
||
Windows PowerShell | for ($i = first; $i -le last; $i++) { instructions } | foreach (item in set) { instructions using item } | ||
D | foreach (i; first ... last) { instructions } | foreach («type» item; set) { instructions } | ||
Go | for condition { instructions } | for i := first; i <= last; i++ { instructions } | for key, item := range set { instructions } | |
Perl | while (condition) { instructions } or until (notcondition) { instructions } |
do { instructions } while (condition) or do { instructions } until (notcondition) |
for«each» «$i» (0 .. N-1) { instructions } or for ($i = first; $i <= last; $i++) { instructions } |
for«each» «$item» (set) { instructions } |
Perl 6 | while condition { instructions } or until notcondition { instructions } |
repeat { instructions } while condition or repeat { instructions } until notcondition |
for first..last -> $i { instructions } or loop ($i = first; $i <= last; $i++) { instructions } |
for set« -> $item» { instructions } |
Ruby | while condition
or until notcondition
|
begin
or begin
|
for i in first...last
or first.upto(last-1) { |
for item in set
or set.each { |
Common Lisp | (loop
(do (notcondition)
|
(loop
|
(loop
(dotimes (i N)
(do ((i first (1+ i))) ((>= i last))
|
(loop
(dolist (item set)
(mapc function list) or (map |
Scheme | (do (notcondition) instructions) or (let loop (if condition (begin instructions (loop)))) |
(let loop (instructions (if condition (loop)))) | (do ((i first (+ i 1))) ((>= i last)) instructions) or (let loop ((i first)) (if (< i last) (begin instructions (loop (+ i 1))))) |
(for-each (lambda (item) instructions) list) |
Pascal | while condition do begin
|
repeat
|
for i := first «step 1» to last do begin
|
|
Visual Basic | Do While condition
or Do Until notcondition
|
Do
or Do
|
For i = first To last «Step 1»
|
For Each item In set
|
Visual Basic .NET | For i «As type» = first To last «Step 1»
|
For Each item As type In set
|
||
Python | while condition : instructions «else: instructions» |
for i in range(first, last): instructions «else: instructions» |
for item in set: instructions «else: instructions» |
|
S-Lang | while (condition) { instructions } «then optional-block» | do { instructions } while (condition) «then optional-block» | for (i = first; i < last; i++) { instructions } «then optional-block» | foreach item(set) «using (what)» { instructions } «then optional-block» |
Fortran | DO WHILE (condition)
|
DO
IF (condition) EXIT |
DO I = first,last
|
|
Forth | BEGIN « instructions » condition WHILE instructions REPEAT | BEGIN instructions condition UNTIL | limit start DO instructions LOOP | |
OCaml | while condition do instructions done | for i = first to last-1 do instructions done | Array.iter (fun item -> instructions) array List.iter (fun item -> instructions) list |
|
F# | while condition do instructions |
for i = first to last-1 do instructions |
for item in set do instructions or Seq.iter (fun item -> instructions) set |
|
Standard ML | while condition do ( instructions ) | colspan=2 | Array.app (fn item => instructions) array app (fn item => instructions) list |
|
Haskell (GHC) | colspan=2 | Control.Monad.forM_ [0..N-1] (\i -> do instructions) | Control.Monad.forM_ list (\item -> do instructions) | |
Eiffel | from
|
"step n" is used to change the loop interval. If "step" is omitted, then the loop interval is 1.
ExceptionsException handlingException handling is a programming language construct or computer hardware mechanism designed to handle the occurrence of exceptions, special conditions that change the normal flow of program execution....
throw | handler | assertion | |
---|---|---|---|
C (C99) | longjmp(state, exception); | switch (setjmp(state)) { case 0: instructions break; case exception: instructions ... } | assert(condition); |
C++ (STL) | throw exception; | try { instructions } catch «(exception)» { instructions } ... | |
C# | try { instructions } catch «(exception)» { instructions } ... «finally { instructions }» | Debug.Assert(condition); | |
Java | try { instructions } catch (exception) { instructions } ... «finally { instructions }» | assert condition; | |
JavaScript | try { instructions } catch (exception) { instructions } «finally { instructions }» | ||
D | try { instructions } catch (exception) { instructions } ... «finally { instructions }» | assert(condition); | |
PHP | try { instructions } catch (exception) { instructions } ... | assert(condition); | |
S-Lang | try { instructions } catch «exception» { instructions } ... «finally { instructions }» | ||
Windows PowerShell | trap «[exception]» { instructions } ... instructions or try { instructions } catch «[exception]» { instructions } ... «finally { instructions }» | [Debug]::Assert(condition) | |
Objective-C | @throw exception; | @try { instructions } @catch (exception) { instructions } ... «@finally { instructions }» | NSAssert(condition, description); |
Perl | die exception; | eval { instructions }; if ($@) { instructions } | |
Perl 6 | try { instructions CATCH { when exception { instructions } ...}} | ||
Ruby | raise exception | begin
«else
|
|
Common Lisp | (error "exception") or (error (make-condition
|
(handler-case
(handler-bind
|
(assert condition) or (assert condition
(check-type var type) |
Scheme (R6RS) | (raise exception) | (guard (con (condition instructions) ...) instructions) | |
Pascal | raise Exception.Create | try Except on E: exception do begin instructions end; end; | |
Visual Basic | colspan=2 | ||
Visual Basic .NET | Throw exception | Try
«Finally
|
Debug.Assert(condition) |
Python | raise exception | try: instructions except «exception»: instructions ... «else: instructions» «finally: instructions» |
assert condition |
Fortran | colspan=3 | ||
Forth | code THROW | xt CATCH ( code or 0 ) | |
OCaml | raise exception | try expression with pattern -> expression ... | assert condition |
F# | try expression with pattern -> expression ... or try expression finally expression |
||
Standard ML | raise exception «arg» | expression handle pattern => expression ... | |
Haskell (GHC) | throw exception or throwError expression |
catch tryExpression catchExpression or catchError tryExpression catchExpression |
assert condition expression |
Common Lisp allows
with-simple-restart
, restart-case
and restart-bind
to define restarts for use with invoke-restart
. Unhandled conditions may cause the implementation to show a restarts menu to the user before unwinding the stack.Other control flow statements
exit block(break) | continue | label Label (programming language) A label in a programming language is a sequence of characters that identifies a location within source code. In most languages labels take the form of an identifier, often followed by a punctuation character . In many high level programming languages the purpose of a label is to act as the... |
branch (goto Goto goto is a statement found in many computer programming languages. It is a combination of the English words go and to. It performs a one-way transfer of control to another line of code; in contrast a function call normally returns control... ) |
return value from generator | |
---|---|---|---|---|---|
ALGOL 68 | value exit; ... | do statements; skip exit; label: statements od | label: ... | go to label; ... goto label; ... label; ... |
yield(value) (Callback Callback (computer science) In computer programming, a callback is a reference to executable code, or a piece of executable code, that is passed as an argument to other code. This allows a lower-level software layer to call a subroutine defined in a higher-level layer.... - example) |
C (C99) | break; | continue; | label: | goto label; | rowspan=4 |
Objective-C | |||||
C++ (STL) | |||||
D | |||||
C# | yield return value; | ||||
Java | break «label»; | continue «label»; | rowspan=2 | ||
JavaScript | yield value«;» | ||||
PHP | break «levels»; | continue «levels»; | goto label; | ||
Perl | last «label»; | next «label»; | |||
Perl 6 | |||||
Go | break «label» | continue «label» | goto label | ||
Common Lisp | (return) or (return-from block) or (loop-finish) |
(tagbody tag
|
(go tag) | ||
Scheme | |||||
Pascal(ISO) | colspan=2 | label: | goto label; | rowspan=4 | |
Pascal(FPC) | break; | continue; | |||
Visual Basic | Exit block | label: | GoTo label | ||
Visual Basic .NET | Continue block | ||||
Python | break | continue | colspan=2 | yield value | |
S-Lang | break; | continue; | |||
Fortran | EXIT | CYCLE | label | GOTO label | |
Ruby | break | next | |||
Windows PowerShell | break« label» | continue | |||
OCaml | rowspan=4 colspan=4 | ||||
F# | |||||
Standard ML | |||||
Haskell (GHC) |
Pascal has declaration blocks. See Comparison of programming languages (basic instructions)#Functions.
label must be a number between 1 and 99999.
FunctionsSubroutineIn computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
See reflectionReflection (computer science)
In computer science, reflection is the process by which a computer program can observe and modify its own structure and behavior at runtime....
for calling and declaring functions by strings.
calling a function | basic/void function | value-returning function | required main function | |
---|---|---|---|---|
ALGOL 68 ALGOL 68 ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a... |
foo«(parameters)»; | proc foo = «(parameters)» void Void type The void type, in several programming languages derived from C and Algol68, is the type for the result of a function that returns normally, but does not provide a result value to its caller. Usually such functions are called for their side effects, such as performing some task or writing to their... : ( instructions ); |
proc foo = «(parameters)» rettype: ( instructions ...; retvalue ); | |
C (C99) | foo(«parameters») | void Void type The void type, in several programming languages derived from C and Algol68, is the type for the result of a function that returns normally, but does not provide a result value to its caller. Usually such functions are called for their side effects, such as performing some task or writing to their... foo(«parameters») { instructions } |
type foo(«parameters») { instructions ... return value; } | «global declarations» int main(«int argc, char *argv[]») {
|
Objective-C | ||||
C++ (STL) | ||||
C# | static void Main(«string[] args») { instructions } or static int Main(«string[] args») { instructions } |
|||
Java | public static void main(String[] args) { instructions } or public static void main(String... Variadic function In computer programming, a variadic function is a function of indefinite arity, i.e., one which accepts a variable number of arguments. Support for variadic functions differs widely among programming languages.... args) { instructions } |
|||
D | int main(«char[][] args») { instructions} or int main(«string[] args») { instructions} or void main(«char[][] args») { instructions} or void main(«string[] args») { instructions} |
|||
JavaScript | function foo(«parameters») { instructions } or var foo = function («parameters») {instructions } or var foo = new Function («"parameter", ... ,"last parameter"» "instructions"); |
function foo(«parameters») { instructions ... return value; } | ||
Go | func foo(«parameters») { instructions } | func foo(«parameters») type { instructions ... return value } | func main { instructions } | |
Common Lisp | (foo «parameters») | (defun Defun defun is a macro in the Lisp family of programming languages that defines a function in the global environment that uses the form:... foo («parameters»)
(setf (symbol-function
|
(defun Defun defun is a macro in the Lisp family of programming languages that defines a function in the global environment that uses the form:... foo («parameters»)
|
rowspan=2 |
Scheme | (define (foo parameters) instructions) or (define foo (lambda Anonymous function In programming language theory, an anonymous function is a function defined, and possibly called, without being bound to an identifier. Anonymous functions are convenient to pass as an argument to a higher-order function and are ubiquitous in languages with first-class functions such as Haskell... (parameters) instructions)) |
(define (foo parameters) instructions... return_value) or (define foo (lambda Anonymous function In programming language theory, an anonymous function is a function defined, and possibly called, without being bound to an identifier. Anonymous functions are convenient to pass as an argument to a higher-order function and are ubiquitous in languages with first-class functions such as Haskell... (parameters) instructions... return_value)) |
||
Pascal | foo«(parameters)» | procedure foo«(parameters)»; «forward;» «label
«const
«type
«var
«local function declarations» begin
|
function foo«(parameters)»: type; «forward;» «label
«const
«type
«var
«local function declarations» begin
foo := valueend; |
program name; «label
«const
«type
«var
«function declarations» begin
|
Visual Basic | Foo(«parameters») | Sub Foo(«parameters»)
|
Function Foo(«parameters») As type
|
Sub Main
|
Visual Basic .NET | Function Foo(«parameters») As type
|
Sub Main(«ByVal CmdArgs As String»)
or Function Main(«ByVal CmdArgs As String») As Integer
|
||
Python | foo(«parameters») | def foo(«parameters»): instructions |
def foo(«parameters»): instructions return value |
|
S-Lang | foo(«parameters» «;qualifiers») | define foo («parameters») { instructions } | define foo («parameters») { instructions ... return value; } | public define slsh_main { instructions } |
Fortran | foo («arguments») CALL sub_foo («arguments») |
SUBROUTINE sub_foo («arguments»)
|
type FUNCTION foo («arguments»)
... foo = value |
PROGRAM main
|
Forth | «parameters» FOO | : FOO « stack effect comment: ( before -- ) »
|
: FOO « stack effect comment: ( before -- after ) »
|
|
PHP | foo(«parameters») | function foo(«parameters») { instructions } | function foo(«parameters») { instructions ... return value; } | rowspan=6 |
Perl | foo(«parameters») or &foo«(parameters)» |
sub foo { «my (parameters) = @_;» instructions } | sub foo { «my (parameters) = @_;» instructions... «return» value; } | |
Perl 6 | foo(«parameters») or &foo«(parameters)» |
«multi »sub foo(parameters) { instructions } | «our «type» »«multi »sub foo(parameters) { instructions... «return» value; } | |
Ruby | foo«(parameters)» | def foo«(parameters)»
|
def foo«(parameters)»
« |
|
Windows PowerShell | foo« parameters» | function foo «(parameters)» { instructions }; or function foo { «param(parameters)» instructions } |
function foo «(parameters)» { instructions … return value }; or function foo { «param(parameters)» instructions … return value } |
|
OCaml | foo parameters | let «rec» foo parameters = instructions | let «rec» foo parameters = instructions... return_value | |
F# | [ |
|||
Standard ML | fun foo parameters = ( instructions ) | fun foo parameters = ( instructions... return_value ) | ||
Haskell | foo parameters = do instructions |
foo parameters = return_value or foo parameters = do instructions return value |
«main :: IO » main = do instructions |
|
Eiffel | foo («parameters») | foo («parameters»)
|
foo («parameters»): type
|
Pascal requires "forward;" for forward declarations.
Eiffel allows the specification of an application's root class and feature.
In Fortran, function/subroutine parameters are called arguments (since PARAMETER is a language keyword); the CALL keyword is required for subroutines.
Type conversionType conversionIn computer science, type conversion, typecasting, and coercion are different ways of, implicitly or explicitly, changing an entity of one data type into another. This is done to take advantage of certain features of type hierarchies or type representations...
s
Where string is a signed decimal number:
string to integer | string to long integer | string to floating point | integer to string | floating point to string | |
---|---|---|---|---|---|
ALGOL 68 ALGOL 68 ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a... with general, and then specific formats |
With prior declarations and association of: string buf := "12345678.9012e34 "; file proxy; associate(proxy, buf); | ||||
get(proxy, ivar); | get(proxy, livar); | get(proxy, rvar); | put(proxy, ival); | put(proxy, rval); | |
getf(proxy, ($g$, ivar)); or getf(proxy, ($dddd$, ivar)); |
getf(proxy, ($g$, livar)); or getf(proxy, ($8d$, livar)); |
getf(proxy, ($g$, rvar)); or getf(proxy, ($8d.4dE2d$, rvar)); |
putf(proxy, ($g$, ival)); or putf(proxy, ($4d$, ival)); |
putf(proxy, ($g(width, places, exp)$, rval)); or putf(proxy, ($8d.4dE2d$, rval)); etc. |
|
C (C99) | integer = atoi(string); | long = atol(string); | float = atof(string); | sprintf(string, "%i", integer); | sprintf(string, "%f", float); |
Objective-C | integer = [string intValue]; | long = [string longLongValue]; | float = [string doubleValue]; | string = [NSString stringWithFormat:@"%i", integer]; | string = [NSString stringWithFormat:@"%f", float]; |
C++ (STL) | «std::»istringstream(string) >> number; | «std::»ostringstream o; o << number; string = o.str; | |||
C++11 | integer = «std::»stoi(string); | long = «std::»stol(string); | float = «std::»stof(string); double = «std::»stod(string); |
string = «std::»to_string(number); | |
C# | integer = int.Parse(string); | long = long.Parse(string); | float = float.Parse(string); or double = double.Parse(string); |
string = number.ToString; | |
D | integer = std.conv.to!int(string) | long = std.conv.to!long(string) | float = std.conv.to!float(string) or double = std.conv.to!double(string) |
string = std.conv.to!string(number) | |
Java | integer = Integer.parseInt(string); | long = Long.parseLong(string); | float = Float.parseFloat(string); or double = Double.parseDouble(string); |
string = Integer.toString(integer); | string = Float.toString(float); or string = Double.toString(double); |
JavaScript | integer = parseInt(string); | float = parseFloat(string); or float = new Number (string) or float = Number (string) or float = string*1; |
string = number.toString ; or string = new String (number); or string = String (number); or string = number+""; |
||
Go | integer, error = strconv.Atoi(string) | long, error = strconv.Atoi64(string) | float, error = strconv.Atof(string) | string = strconv.Itoa(integer) or string = fmt.Sprint(integer) |
string = strconv.Ftoa(float) or string = fmt.Sprint(float) |
Common Lisp | (setf integer (parse-integer string)) | (setf float (read-from-string string)) | (setf string (princ-to-string number)) | ||
Scheme | (define number (string->number string)) | (define string (number->string number)) | |||
Pascal | integer := StrToInt(string); | float := StrToFloat(string); | string := IntToStr(integer); | string := FloatToStr(float); | |
Visual Basic | integer = CInt(string) | long = CLng(string) | float = CSng(string) or double = CDbl(string) |
string = CStr(number) | |
Visual Basic .NET | |||||
Python | integer = int(string) | long = long(string) | float = float(string) | string = str(number) | |
S-Lang | integer = atoi(string); | long = atol(string); | float = atof(string); | string = string(number); | |
Fortran | READ(string,format) number | WRITE(string,format) number | |||
PHP | integer = intval(string); or integer = (int)string; |
float = floatval(string); or float = (float)string; |
string = "number"; or string = strval(number); or string = (string)number; |
||
Perl | number = 0 + string; | string = "number"; | |||
Perl 6 | number = +string; | string = ~number; | |||
Ruby | integer = string.to_i or integer = Integer(string) |
float = string.to_f or float = Float(string) |
string = number.to_s | ||
Windows PowerShell | integer = [int]string | long = [long]string | float = [float]string | string = [string]number; or string = "number"; or string = (number).ToString |
|
OCaml | let integer = int_of_string string | let float = float_of_string string | let string = string_of_int integer | let string = string_of_float float | |
F# | let integer = int string | let integer = int64 string | let float = float string | let string = string number | |
Standard ML | val integer = Int.fromString string | val float = Real.fromString string | val string = Int.toString integer | val string = Real.toString float | |
Haskell (GHC) | number = read string | string = show number |
JavaScript only uses floating point numbers so there are some technicalities.
Perl doesn't have separate types. Strings and numbers are interchangeable.
Standard Stream I/OStandard streamsIn Unix and Unix-like operating systems , as well as certain programming language interfaces, the standard streams are preconnected input and output channels between a computer program and its environment when it begins execution...
read from | write to | ||
---|---|---|---|
stdin | stdout | stderr | |
ALGOL 68 ALGOL 68 ALGOL 68 isan imperative computerprogramming language that was conceived as a successor to theALGOL 60 programming language, designed with the goal of a... |
readf(($format$, x)); or getf(stand in, ($format$, x)); |
printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... (($format$, x)); or putf(stand out, ($format$, x)); |
putf(stand error, ($format$, x)); |
C (C99) | scanf Scanf Scanf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for reading a string into an arbitrary number of varied data type parameter... (format, &x); or fscanf(stdin, format, &x); |
printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... ( format, x); or fprintf(stdout, format, x); |
fprintf(stderr, format, x ); |
Objective-C | data = SFileHandle fileHandleWithStandardInput] readDataToEndOfFile]; | SFileHandle fileHandleWithStandardOutput] writeData:data]; | SFileHandle fileHandleWithStandardError] writeData:data]; |
C++ | «std::»cin Iostream iostream is a header file which is used for input/output in the C++ programming language. It is part of the C++ standard library. The name stands for Input/Output Stream. In C++ and its predecessor, the C programming language, there is no special syntax for streaming data input or output. Instead,... >> x; or «std::»getline(«std::»cin, str); |
«std::»cout Iostream iostream is a header file which is used for input/output in the C++ programming language. It is part of the C++ standard library. The name stands for Input/Output Stream. In C++ and its predecessor, the C programming language, there is no special syntax for streaming data input or output. Instead,... << x; |
«std::»cerr Iostream iostream is a header file which is used for input/output in the C++ programming language. It is part of the C++ standard library. The name stands for Input/Output Stream. In C++ and its predecessor, the C programming language, there is no special syntax for streaming data input or output. Instead,... << x; or «std::»clog Iostream iostream is a header file which is used for input/output in the C++ programming language. It is part of the C++ standard library. The name stands for Input/Output Stream. In C++ and its predecessor, the C programming language, there is no special syntax for streaming data input or output. Instead,... << x; |
C# | x = Console.Read; or x = Console.ReadLine; |
Console.Write(«format, »x); or Console.WriteLine(«format, »x); |
Console.Error.Write(«format, »x); or Console.Error.WriteLine(«format, »x); |
D | x = std.stdio.readln | std.stdio.write(x) or std.stdio.writeln(x) or std.stdio.writef(format, x) or std.stdio.writefln(format, x) |
stderr.write(x) or stderr.writeln(x) or std.stdio.writef(stderr, format, x) or std.stdio.writefln(stderr, format, x) |
Java | x = System.in.read; or x = new Scanner(System.in).nextInt; or x = new Scanner(System.in).nextLine; |
System.out.print(x); or System.out.printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... (format, x); or System.out.println(x); |
System.err.print(x); or System.err.printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... (format, x); or System.err.println(x); |
Go | fmt.Scan(&x) or fmt.Scanf Scanf Scanf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for reading a string into an arbitrary number of varied data type parameter... (format, &x) or x = bufio.NewReader(os.Stdin).ReadString('\n') |
fmt.Println(x) or fmt.Printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... (format, x) |
fmt.Fprintln(os.Stderr, x) or fmt.Fprintf(os.Stderr, format, x) |
JavaScript Web Browser implementation Client-side JavaScript Client-side JavaScript is JavaScript that runs on the client-side. While JavaScript was originally created to run this way, the term was coined because the language is no longer limited to just client-side, since server-side JavaScript is now available.-Environment:The most common Internet media... |
document.write(x) | ||
JavaScript Active Server Pages Active Server Pages Active Server Pages , also known as Classic ASP or ASP Classic, was Microsoft's first server-side script engine for dynamically-generated Web pages. Initially released as an add-on to Internet Information Services via the Windows NT 4.0 Option Pack Active Server Pages (ASP), also known as Classic... |
Response.Write(x) | ||
JavaScript Windows Script Host Windows Script Host The Microsoft Windows Script Host is an automation technology for Microsoft Windows operating systems that provides scripting capabilities comparable to batch files, but with a greater range of supported features... |
x = WScript.StdIn.Read(chars) or x = WScript.StdIn.ReadLine |
WScript.Echo(x) or WScript.StdOut.Write(x) or WScript.StdOut.WriteLine(x) |
WScript.StdErr.Write(x) or WScript.StdErr.WriteLine(x) |
Common Lisp | (setf x (read-line)) | (princ x) or (format t format x) |
(princ x *error-output*) or (format *error-output* format x) |
Scheme (R6RS) | (define x (read-line)) | (display x) or (format #t format x) |
(display x (current-error-port)) or (format (current-error-port) format x) |
Pascal | read(x); or readln(x); |
write(x); or writeln(x); |
rowspan=2 |
Visual Basic | Input« prompt,» x | Print x or ? x |
|
Visual Basic .NET | x = Console.Read or x = Console.ReadLine |
Console.Write(«format, »x) or Console.WriteLine(«format, »x) |
Console.Error.Write(«format, »x) or Console.Error.WriteLine(«format, »x) |
Python 2.x | x = raw_input(«prompt») | print x or sys.stdout.write(x) |
print >> sys.stderr, x or sys.stderr.write(x) |
Python 3.x | x = input(«prompt») | print(x«, end=""») | print(x«, end=""», file=sys.stderr) |
S-Lang | fgets (&x, stdin) | fputs (x, stdout) | fputs (x, stderr) |
Fortran | READ(*,format) variable names or READ(INPUT_UNIT,format) variable names |
WRITE(*,format) expressions or WRITE(OUTPUT_UNIT,format) expressions |
WRITE(ERROR_UNIT,format) expressions |
Forth | buffer length ACCEPT ( # chars read ) KEY ( char ) |
buffer length TYPE char EMIT |
|
PHP | $x = fgets(STDIN); or $x = fscanf(STDIN, format); |
print x; or echo x; or printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... (format, x); |
fprintf(STDERR, format, x); |
Perl | $x = <>; or $x = |
print x; or printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... format, x; |
print STDERR x; or printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... STDERR format, x; |
Perl 6 | $x = $*IN.get; | x.print or x.say |
x.note or $*ERR.print(x) or $*ERR.say(x) |
Ruby | x = gets | puts x or printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... (format, x) |
$stderr.puts(x) or $stderr.printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... (format, x) |
Windows PowerShell | $x = Read-Host«« -Prompt» text»; or $x = [Console]::Read; or $x = [Console]::ReadLine |
x; or Write-Output x; or echo x |
Write-Error x |
OCaml | let x = read_int or let str = read_line or Scanf.scanf Scanf Scanf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for reading a string into an arbitrary number of varied data type parameter... format (fun x ... -> ...) |
print_int x or print_endline str or Printf.printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... format x ... |
prerr_int x or prerr_endline str or Printf.eprintf format x ... |
F# | let x = System.Console.ReadLine | printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... format x ... or printf Printf Printf format string refers to a control parameter used by a class of functions typically associated with some types of programming languages. The format string specifies a method for rendering an arbitrary number of varied data type parameter into a string... n format x ... |
eprintf format x ... or eprintfn format x ... |
Standard ML | val str = TextIO.inputLIne TextIO.stdIn | print str | TextIO.output (TextIO.stdErr, str) |
Haskell (GHC) | x <- readLn or str <- getLine |
print x or putStrLn str |
hPrint stderr x or hPutStrLn stderr str |
Algol 68 additionally as the "unformatted" transput routines: read, write, get and put.
gets(x) and fgets(x, length, stdin) read unformatted text from stdin. Use of gets is not recommended.
puts(x) and fputs(x, stdout) write unformatted text to stdout.
fputs(x, stderr) writes unformatted text to stderr
INPUT_UNIT, OUTPUT_UNIT, ERROR_UNIT are defined in the ISO_FORTRAN_ENV module.
Reading command-line arguments
Argument values | Argument counts | Program name / Script name | |
---|---|---|---|
C (C99) | argv[n] | argc | first argument |
Objective-C | |||
C++ | |||
C# | args[n] | args.Length | Assembly.GetEntryAssembly.Location; |
Java | args.length | ||
D | first argument | ||
JavaScript Windows Script Host Windows Script Host The Microsoft Windows Script Host is an automation technology for Microsoft Windows operating systems that provides scripting capabilities comparable to batch files, but with a greater range of supported features... implementation |
WScript.Arguments(n) | WScript.Arguments.length | |
Go | os.Args[n] | len(os.Args) | first argument |
Common Lisp | |||
Scheme (R6RS) | (list-ref (command-line) n) | (length (command-line)) | first argument |
Pascal | ParamStr(n) | ParamCount | first argument |
Visual Basic | Command | App.Path | |
Visual Basic .NET | CmdArgs(n) | CmdArgs.Length | [Assembly].GetEntryAssembly.Location |
Python | sys.argv[n] | len(sys.argv) | first argument |
S-Lang | __argv[n] | __argc | first argument |
Fortran | DO i = 1,argc
|
argc = COMMAND_ARGUMENT_COUNT | CALL GET_COMMAND_ARGUMENT (0,progname) |
PHP | $argv[n] | $argc | first argument |
Perl | $ARGV[n] | scalar(@ARGV) | $0 |
Perl 6 | @*ARGS[n] | @*ARGS.elems | $PROGRAM_NAME |
Ruby | ARGV[n] | ARGV.size | $0 |
Windows PowerShell | $args[n] | $args.Length | $MyInvocation.MyCommand.Name |
OCaml | Sys.argv.(n) | Array.length Sys.argv | first argument |
F# | args.[n] | args.Length | Assembly.GetEntryAssembly.Location |
Standard ML | List.nth (CommandLine.arguments , n) | length (CommandLine.arguments ) | CommandLine.name |
Haskell (GHC) | do { args <- System.getArgs; return args !! n } | do { args <- System.getArgs; return length args } | System.getProgName |
Execution of commands
Shell command | Execute program | Replace current program with new executed program Exec (operating system) The exec collection of functions of Unix-like operating systems cause the running process to be completely replaced by the program passed as an argument to the function... |
|
---|---|---|---|
C | system("command"); | execl Exec (operating system) The exec collection of functions of Unix-like operating systems cause the running process to be completely replaced by the program passed as an argument to the function... (path, args); or execv Exec (operating system) The exec collection of functions of Unix-like operating systems cause the running process to be completely replaced by the program passed as an argument to the function... (path, arglist); |
|
C++ | |||
Objective-C | [NSTask launchedTaskWithLaunchPath:(NSString *)path arguments:(NSArray *)arguments]; | ||
C# | System.Diagnostics.Process.Start(path, argstring); | ||
F# | |||
Go | exec.Run(path, argv, envv, dir, exec.DevNull, exec.DevNull, exec.DevNull) | os.Exec(path, argv, envv) | |
Visual Basic | Interaction.Shell(command «, WindowStyle» «, isWaitOnReturn») | ||
Visual Basic .NET | Microsoft.VisualBasic.Interaction.Shell(command «, WindowStyle» «, isWaitOnReturn») | System.Diagnostics.Process.Start(path, argstring) | |
D | std.process.system("command"); | std.process.execv(path, arglist); | |
Java | Runtime.exec(command); or new ProcessBuilder(command).start; |
||
JavaScript Windows Script Host Windows Script Host The Microsoft Windows Script Host is an automation technology for Microsoft Windows operating systems that provides scripting capabilities comparable to batch files, but with a greater range of supported features... implementation |
WScript.CreateObject ("WScript.Shell").Run(command «, WindowStyle» «, isWaitOnReturn»); | ||
Common Lisp | (shell command) | ||
Scheme | (system command) | ||
Pascal | system(command); | ||
OCaml | Sys.command command, Unix.open_process_full command env (stdout, stdin, stderr),... | Unix.create_process prog args new_stdin new_stdout new_stderr, ... | Unix.execv prog args or Unix.execve prog args env |
Standard ML | OS.Process.system command | Unix.execute (path, args) | Posix.Process.exec (path, args) |
Haskell (GHC) | System.system command | System.Process.runProcess path args ... | Posix.Process.executeFile path True args ... |
Perl | system(command) or $output = `command` |
exec(path, args) | |
Ruby | system(command) or output = `command` |
exec(path, args) | |
PHP | system(command) or $output = `command` or exec(command) or passthru(command) |
||
Python | os.system(command) or subprocess.Popen(command) |
os.execv(path, args) | |
S-Lang | system(command) | ||
Fortran | CALL SYSTEM (command, status) or status = SYSTEM (command) |
||
Windows PowerShell | [Diagnostics.Process]::Start(command) | «Invoke-Item »program arg1 arg2 … |
Compiler-dependent extension.